In Python, the default data type for the ‘input’ command is a string.
A string is essentially a collection of characters, which can include letters, symbols, numbers, and more. It’s important to note that when you input numbers using the ‘input’ command, they are stored as characters within a string. This means that you cannot perform mathematical operations on them directly.
Let’s understand strings with a straightforward Python program, ‘strings.py‘:
_name=input("Enter Name :")
print(_name)
When you run this program, you will get the same string that you entered as the output. This showcases the string data type’s role in capturing and displaying text-based information in Python programs
In Python, when we input data using the ‘input’ function, the input is stored as a collection of characters within a string. Each character in the string is assigned a unique position number, starting with zero at the leftmost character and increasing by 1 as we move rightward through the string. This concept is technically known as “string indexing.”
Index number | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
String | p | u | r | u | s | h | o | t | t | a | m |
String indexing allows us to access and manipulate individual characters within a string by referring to their specific positions, making it a fundamental concept in string processing and manipulation
This indexing gives us ability to interact with individual members of the string, for example
_name[0]
this will give us _name[0] = p
We can also extract part of the string, for example, if we want to assign the nickname as the first four letters of the name,
_name[0:4]
this will give us _name[0:4] = puru
If you observe, the ‘4th’ index member of the string is ‘s’.
However, when we extract [0:4], ‘s’ was not included in the result.
This is because the selection process excludes the upper-bound value. When we extract [0:4], it retrieves characters at indices [0, 1, 2, and 3]. In this case, ‘p,’ ‘u,’ ‘r,’ and ‘u’ are extracted, excluding the character at the upper bound (index 4).
This behavior is a fundamental aspect of Python’s string indexing and slicing
We can also ‘add’ two strings with a straightforward ‘+’ operator. For example
_name=input("Enter Name ")
_middle=input("Enter middle name ")
_fullname=_name+_middle
print(_fullname)
the output we will get for this ‘simple_string.py’ is
An observation, empty spaces need to be defined explicitly, because addition is a simple combination of the strings. So a better command is —
_fullname = _name+" "+_middle
Now, the string ‘_fullname’ has become long. (11 characters + space + 6 characters ), 18 characters long. Suppose, we are required to extract the last four letters ‘xman’ , going by usual indexing (left to right), it will be a long count. To make things easy, python uses negative indexing from right to left, as shown below —
Index | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 |
String | p | u | r | u | s | h | o | t | t | a | m | l | a | x | m | a | n | |
Reverse | -18 | -17 | -16 | -15 | -14 | -13 | -12 | -11 | -10 | -9 | -8 | -7 | -6 | -5 | -4 | -3 | -2 | -1 |
Thus, to extract ‘xman’ from the full string, we can use _fullname[-4,-1]
However, remember the ‘upper-bound’ exclusion, thus ‘-1’ will get excluded and our output will be
_fullname[-4, -1] = xma
to get around this, we will not give the upper-bound
_fullname[-4 : ] = xman
Let’s combine and illustrate our string operations in a ‘string_indexing.py’
Moving further, it’s important to consider that the output of our program should adhere to English language rules, which typically require the first letter of a proper noun (such as a name) to be in uppercase (capitalized). While a typical user might provide input in lowercase, our program should be able to correct such basic mistakes. To achieve this, Python provides string functions.
For instance, to convert the first letter of a string to uppercase, we can use the ‘capitalize()’ function. The typical syntax for using functions is as follows:
string_name.function_name(arguments_if_any)
In the case of the ‘capitalize()’ function, it can be implemented for the input string as follows:
_name.capitalize()
Let’s combine what we have learnt so far into a new program ‘strings_functions.py’
In the program, you’ll notice an interesting operation. After using the ‘capitalize()’ function, we could have created a new variable to store the capitalized name
_name = input("Enter First Name: ")
_capitalized_name = _name.capitalize()
However, creating too many intermediate variables can lead to confusion and is unnecessary if we have no further use for the original variable. Therefore, we use the technique of reassigning the variable to rewrite itself:
_name = _name.capitalize()
This ability to rewrite the same variable with its modified value is extremely useful. It not only reduces the number of variables but also has broader applications, including creating loops and more, which we will explore in later chapters
Exercise
- Create a python program, which will ask the user to input — name, height, and weight, and give the below output
Issac H 140 cm W 50 kg
- Create a python program prompts the user for a word, and prints out the number of letters in the word
Input — orange, resultant, narrow, booth, wisdom
- Create a python program that prompts a user to enter his favorite football team, prints the following output
ARSENAL are a good football team
- An automated program is used to create user names for students. The username will be the first three letters of the first name, followed by the last two letters of the last name.
- Modify previous program (no 4), such that, the first three letters of the username are in uppercase, and the last two are in lower case
Hint: function to count the number of letters is variable name•count( ). To convert all letters into upper case the function is variable name•upper( ) and similarly for lowercase is variable name•lower( )
Lists
While the ‘string’ data type exclusively handles characters, real-world applications often require interactions between different data types, such as student names, roll numbers, birth dates, exam grades, and attendance percentages. To handle this diversity of data types, we have ‘lists’ in Python.
Unlike ‘strings,’ ‘lists’ use square brackets [ ] to define their elements. For example:
_information = ["P L Deshpande", 1919, "November", 8, "Mumbai"]
so if we look at index of _information, we will have
Index No | 0 | 1 | 2 | 3 | 4 |
List | P L Deshpande | 1919 | November | 8 | Mumbai |
Data type | String | Integer | String | Integer | String |
Reverse | -5 | -4 | -3 | -2 | -1 |
Similar to how we access portions of a string using indexing, we can access elements of a list in Python. For example, in the list ‘_information,’ ‘_information[2]’ would yield ‘November.’
Now, using these concepts, let’s create a program that implements lists. We’ll call it ‘basic_list.py.’ This program will help us understand how to work with lists in Python effectively.
Much like strings, lists in Python come equipped with a variety of functions, including handy ones like count()
and append()
. We’ll explore these functions in greater detail as we advance in our programming journey.
Moreover, in the chapters to come, we’ll delve into more complex ideas, such as lists within lists. This opens up exciting possibilities for organizing and structuring data.
Think of lists as your virtual file cabinets, where you can systematically arrange different data types with specifically designated positions. This orderly and versatile approach allows you to efficiently store and manage a diverse array of information in your Python programs.
Dictionaries
In our previous program, ‘basic_list.py,’ prompting the user to enter the month in words can feel counter-intuitive. Once we ask the user to input the date as a number, logically, the month input should also be in numeric form.
Analyzing this further, we have 12 months in a year, which constitutes a limited dataset. Therefore, we should be able to link a month to its corresponding number. This is where ‘dictionaries’ come into play.
Dictionaries in Python are defined using curly braces { }. For example:
_calendar = {1: "January", 2: "February"}
In this dictionary, the number ‘1’ corresponds to ‘January,’ and the number ‘2’ corresponds to ‘February.’
Dictionaries don’t have indexing in the traditional sense because each member has a corresponding member. To access a particular value, you only need to provide the key, and Python will return the corresponding value:
_calendar[2]
This command will search for the value ‘2’ in the dictionary and, when found, will retrieve the corresponding value, which, in this case, is ‘February.’ Dictionaries are a powerful data structure for mapping one set of values to another in Python
Let’s understand this using an example — ‘dictionary_basic.py’
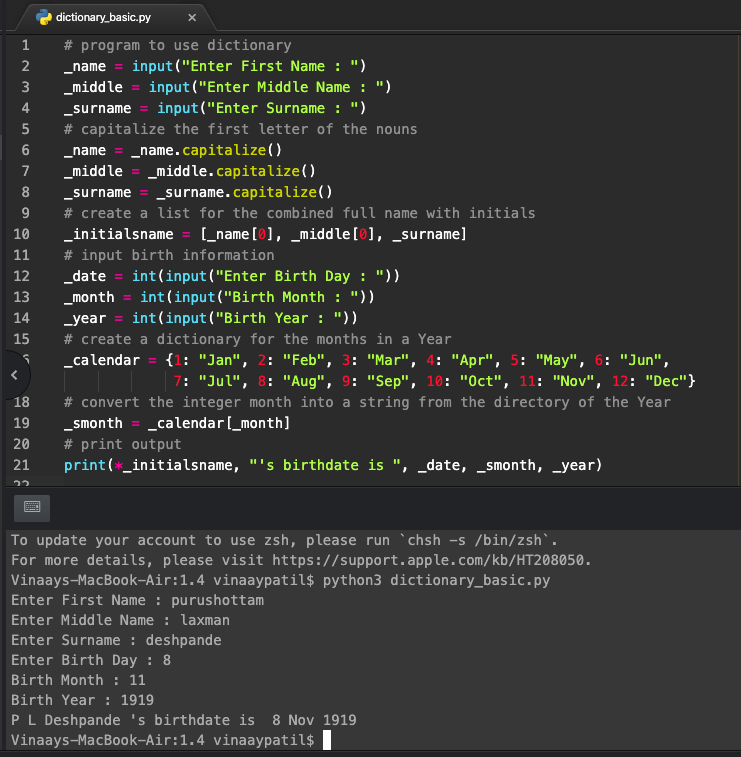
In the above program, we used a specific syntax, ‘*’
The command to print the entire list is print(*list_name)
. In the program, this syntax is used as print(*initialsname)
.
It’s essential to understand that a dictionary is a correspondence between any two data types. In the program, the integer ‘1’ corresponds to the string ‘January’. However, correspondence can exist between any two data types. For instance:
_information = {"Name": "Hawking", "Birth Year": 1942, "Birth Month": "January"}
In this dictionary, “Name” corresponds to “Hawking,” “Birth Year” corresponds to 1942, and “Birth Month” corresponds to “January.” So, if we call _information["Birth Year"]
, we will get the value 1942.
It’s crucial to note that correspondence in dictionaries is one-way, from left to right. This means that if we search for “Birth Year,” we’ll get 1942. However, attempting a reverse search by looking for “1942” to find “Birth Year” will result in an error.”
Exercise
- Create a directory data named ‘_information’ for a student, which stores the student’s roll number, student’s name, and grade level. Prompt for inputs and get the following output
Racheal Roll No 14 Grade A
- Create a directory of roll numbers for the following students, 1-Newton, 2-Galileo, 3-Planck, 4-Heisenberg, 5-Bohr. And then when the user inputs a roll number, the name of the student is returned
Enter roll no5 Student name is Bohr
- Create a program to prompt for the First name and last name of a person. Using this data create the following information points, User Name = First three letters of first name + Last two letters of the last name, Password = First letter of the last name in upper case + count of letters in the name + last letter of first name + count of the letters of the last name. The following output is desired for the name ‘Albert Einstein’
Enter Name: Albert Username is Albin
- Create a python program that can store name, account number, and account balance, and when the user inputs the account number, it reveals the account balance.
Enter Account Number1409 Account Balance is 23000
- In the previous program, input two account details (Einstein, Acc no 1409, AccBalance 23000) and (Newton, Acc no 1211, Acc Balance 9500). Use the essence of exercise problem 3 to create unique user names and passwords for each of them. Then create a program that asks for the user name, and it gives out the account number.
Summary
In this chapter, we explored the fundamental concepts of Python’s string data type and introduced the idea of string indexing. We learned that when you input data using the ‘input’ command, it’s stored as a collection of characters within a string. Each character in the string is assigned a unique position number, starting with zero at the leftmost character and increasing as you move rightward. We delved into string indexing, which allows us to interact with individual characters within a string, and slicing, which enables us to extract specific portions of a string.
We also discussed string operations such as concatenation using the ‘+’ operator. It’s essential to note that strings are case-sensitive, and the default data type for ‘input’ is a string. To adhere to English language rules, Python provides string functions like ‘capitalize()’ to convert the first letter of a string to uppercase.
The chapter presented several exercises to practice these concepts, which included creating programs to format and process user input, working with string indices, and utilizing string functions.
We also touched on the use of lists, which are essential for handling different data types and organizing information. Lists allow you to store and manage diverse data in an orderly manner, just like a virtual file cabinet.
Lastly, we introduced dictionaries as a way to create correspondences between different data types. Dictionaries are powerful for mapping one set of values to another, allowing you to create meaningful relationships in your Python programs.
Throughout the chapter, you had the opportunity to practice and apply these concepts through exercises that gradually increased in complexity. These exercises provided hands-on experience in working with strings, lists, and dictionaries, and they set the stage for more advanced programming concepts in the chapters to come.
[…] Prev Chapter Course Homepage Next Chapter […]